Calculate Your Age in Seconds
- Home
- portfolio
- Development
- Calculate Your Age in Seconds
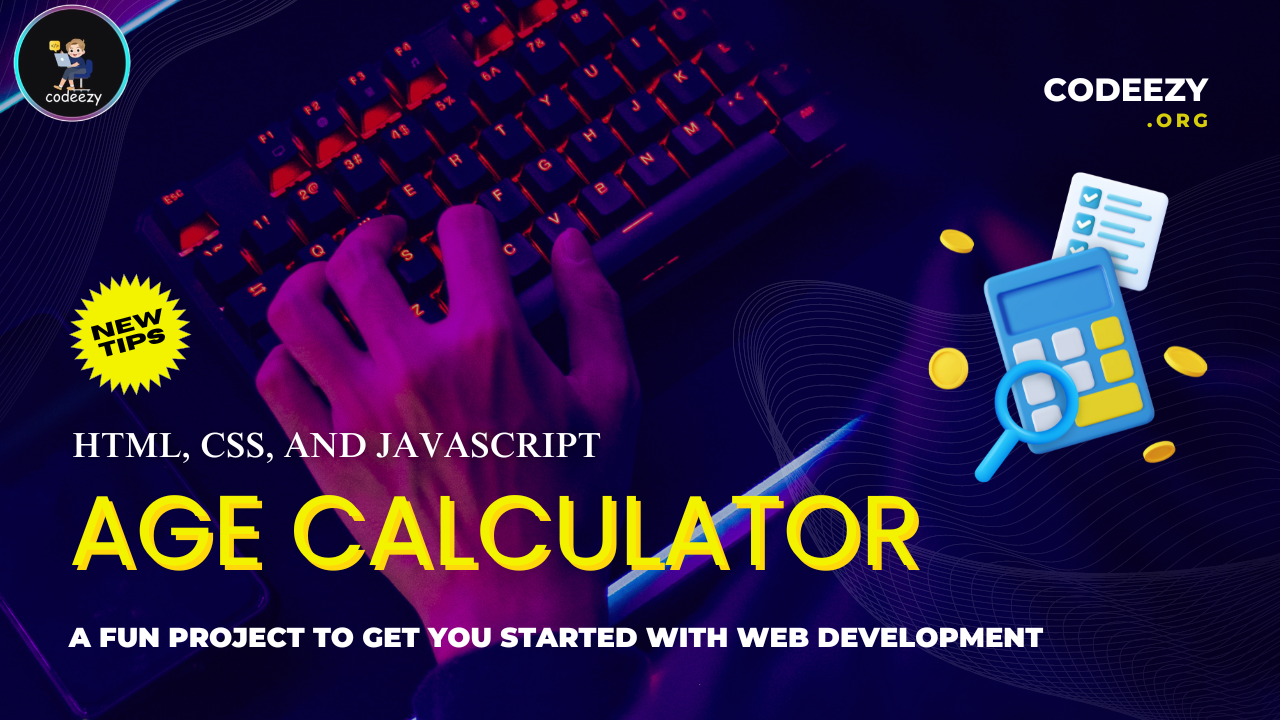
Building an Age Calculator with HTML, CSS, and JavaScript: A Step-by-Step Guide
A Fun Project to Get You Started with Web Development
Hey there, Codeezy community!
Are you new to web development and looking for a fun project to get started with? Or maybe you’re just looking for a quick and easy project to build your portfolio? Either way, you’re in the right place!
In this post, we’ll be building a simple age calculator using HTML, CSS, and JavaScript. This project is perfect for beginners, and it’s a great way to practice your coding skills.
What is an Age Calculator?
An age calculator is a simple web application that takes a user’s date of birth as input and calculates their age in real-time. It’s a fun and useful tool that can be used for a variety of purposes, from calculating your own age to determining the age of a friend or family member.
How Does it Work?
Our age calculator will work by taking the user’s date of birth as input and using JavaScript to calculate their age. We’ll use the Date
object in JavaScript to get the current date and time, and then subtract the user’s birthdate from the current date to get their age.
Let’s Get Started!
To build our age calculator, we’ll need to create three files: index.html
, style.css
, and script.js
. We’ll start with the HTML file, which will contain the structure of our web page.
Source code 👇👇
Age Calculator
Codeezy.org
Enter the Date of birth (MM/DD/YYYY)
Next, we’ll add some styles to our web page using CSS.
*{
margin:0;
padding:0;
box-sizing:border-box;
font-family:'Times New Roman', Times, serif;
}
.container{
display:flex;
width:600px;
margin:auto;
margin-top:10%;
margin-bottom:10%;
align-items:center;
justify-content:center;
flex-direction:column;
background-color:rgb(33, 3, 233);
box-shadow:8px 8px rgb(0, 0, 0);
color:white;
padding:5% 0%;
border-radius: 20px;
}
#currDate{
font-size:40px;
margin:20px;
font-weight:bold;
}
input{
font-size:20px;
padding:15px;
margin:20px;
text-align:center;
border-radius:20px;
border:1px solid rgb(6, 243, 65);
cursor:pointer;
}
button{
font-size:20px;
padding:10px 20px;
border-radius:10px;
border:none;
background-color:rgb(255, 225, 0);
color:black;
margin:20px;
text-transform: uppercase;
font-weight:bold;
cursor:pointer;
}
button:hover{
background-color:white;
color:blue;
}
#displayAge{
display:flex;
align-items:center;
justify-content:center;
width:620px;
height:480px;
background-color:rgb(248, 8, 224);
border-radius:50px;
position:absolute;
top:15%;
left:23%;
visibility: hidden;
}
#age{
color:white;
font-size:50px;
margin:20px;
font-weight:bold;
}
Finally, we’ll add the JavaScript code to calculate the user’s age.
let currDate= document.getElementById("currDate");
let dateOfBirth = document.querySelector("#DOB");
const CalcAge= document.getElementById("CalcAge");
const displayAge= document.getElementById("displayAge");
const Age= document.getElementById("age");
var today = new Date();
currDate.innerText=`Today's Date is : ${today.toLocaleDateString('en-US')}`;
CalcAge.addEventListener("click",()=>{
var birthDate = new Date(dateOfBirth.value);
var age = today.getFullYear() - birthDate.getFullYear();
var m = today.getMonth() - birthDate.getMonth();
if (m < 0 || (m === 0 && today.getDate() < birthDate.getDate())) {
age = age - 1;
}
displayAge.style.visibility="visible";
Age.innerText=`You are ${age} years old.`
});
Output 👇👇
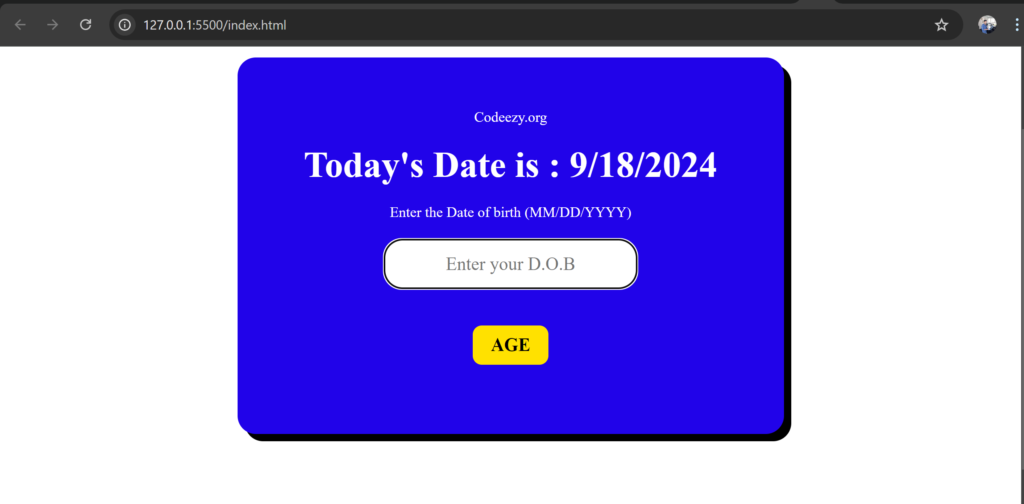
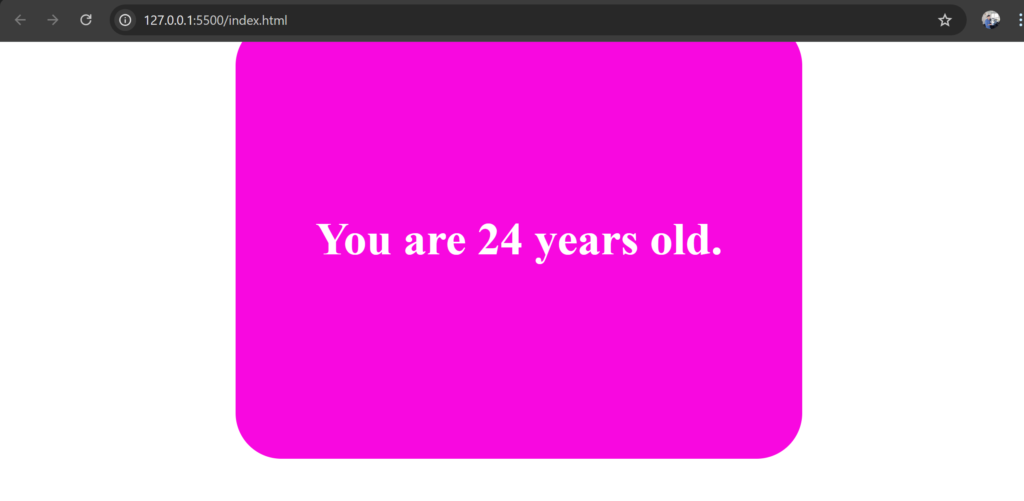
And That’s It!
That’s it for our age calculator project! I hope you had fun building it with me. If you have any questions or need help with any part of the code, feel free to ask in the comments below.
What’s Next?
If you’re looking for more projects to build, be sure to check out our other tutorials and projects on Codeezy. We have a wide range of topics and projects to choose from, from beginner-friendly projects like this one to more advanced projects that will challenge your skills.
Happy Coding!
Thanks for reading, and happy coding!