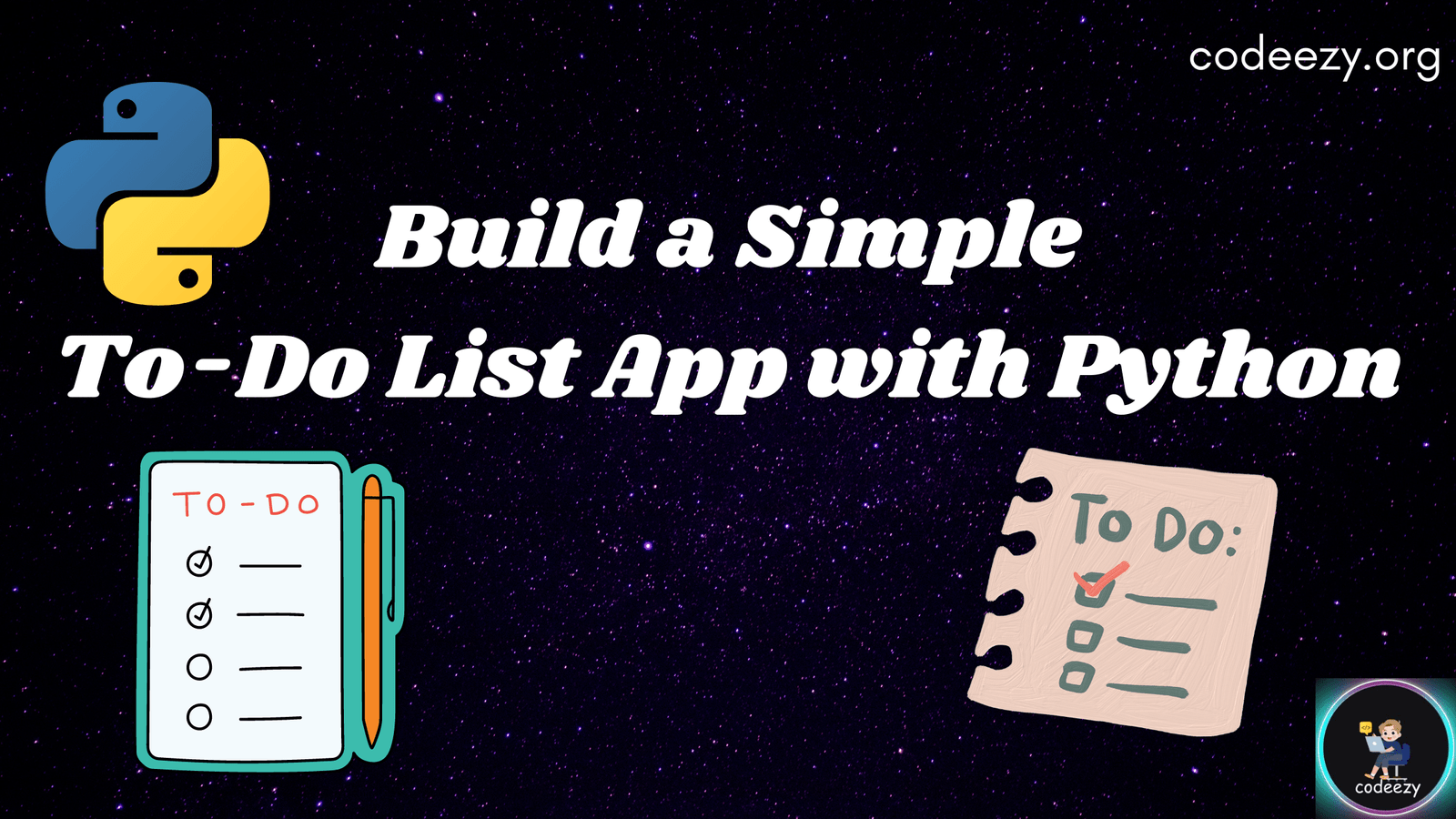
Introduction : To-Do List App with Python
Are you looking to build your first Python project? A To-Do List app is a perfect start. It’s simple, practical, and gives you a hands-on experience with Python’s core functionalities. In this blog post, we’ll walk you through the steps of creating a basic To-Do List app, complete with source code and explanations. Whether you’re a coding newbie or someone looking to practice their skills, this guide has got you covered.
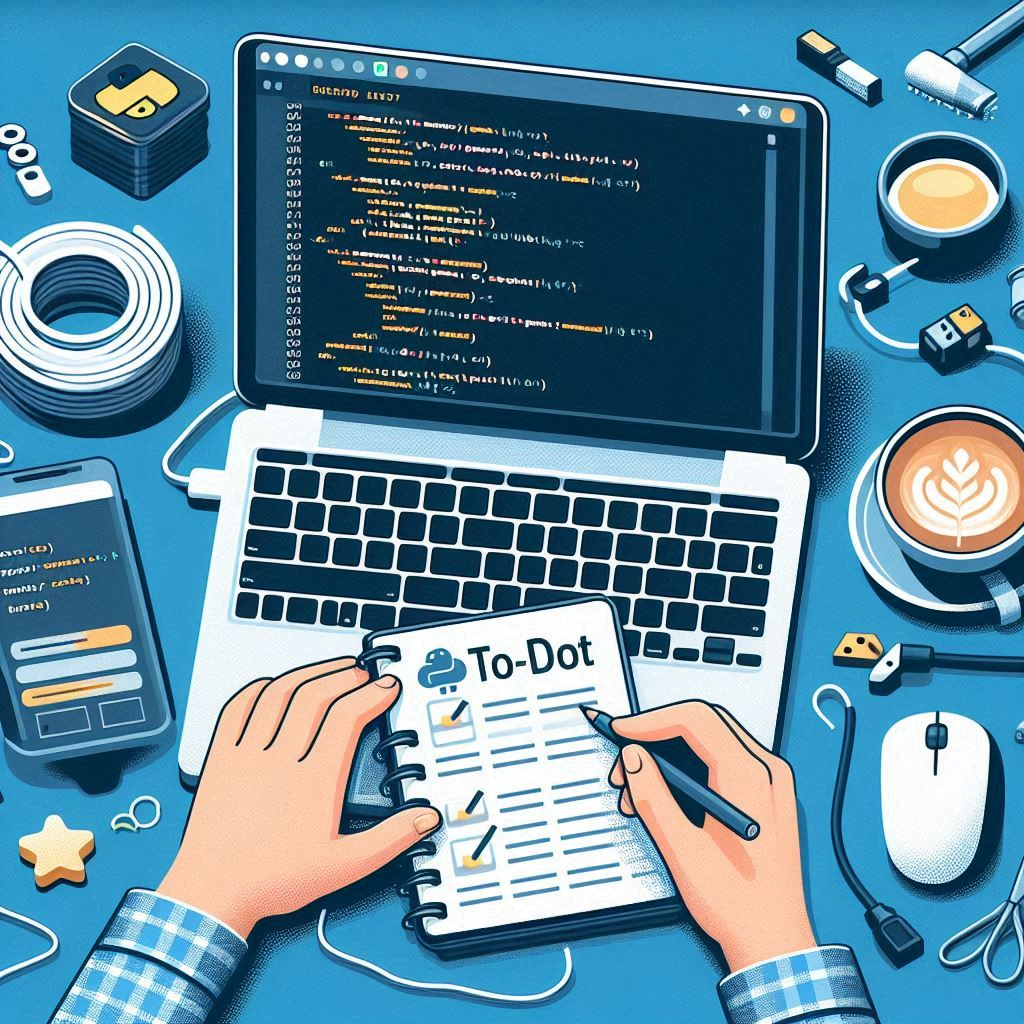
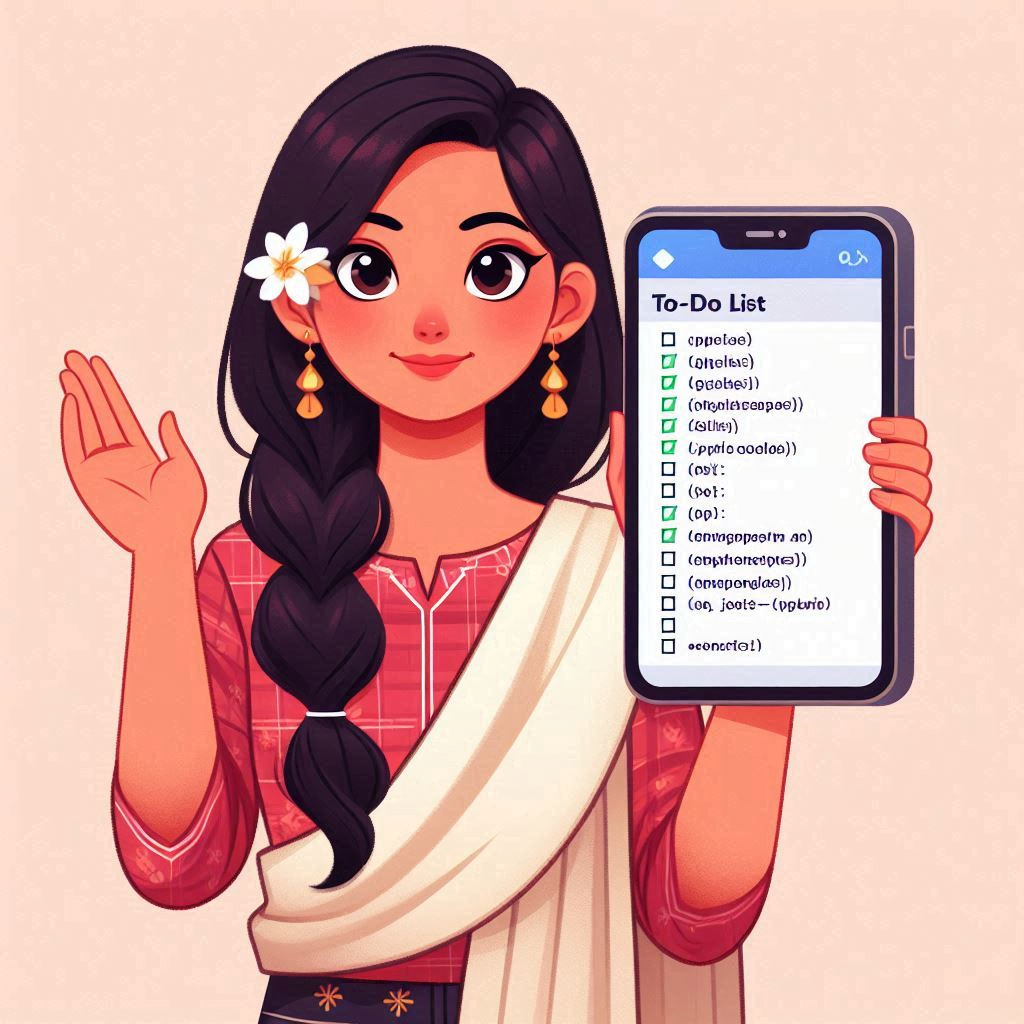
What is a To-Do List App?
A To-Do List app is a simple application where users can manage tasks by adding, deleting, or marking them as complete. This type of app is incredibly useful for organizing your day-to-day activities, setting goals, or managing projects.
Beginner-Friendly: It’s an excellent project for beginners to get comfortable with Python.
Practical Use: You’ll end up with a tool that you can actually use to manage your tasks.
Learn Core Concepts: You’ll get to work with lists, functions, and basic file handling.
Setting Up Your Python Environment
Before you start coding, ensure you have Python installed on your system. You can download the latest version from python.org. Once installed, you can use any code editor or IDE like VS Code, PyCharm, or even a simple text editor.
Step-by-Step Guide to Building the To-Do List App
Step 1: Creating the Basic Structure
Start by creating a Python file called main.py
. This file will house all of your code. Open your code editor and begin by defining a class for your To-Do List App. Here’s the initial setup:
import os
class ToDoList:
def __init__(self, file_name="tasks.txt"):
self.tasks = []
self.file_name = file_name
self.load_tasks()
def load_tasks(self):
"""Load tasks from a file."""
if os.path.exists(self.file_name):
with open(self.file_name, "r") as file:
for line in file:
task, priority = line.strip().split("|")
self.tasks.append({"task": task, "priority": int(priority)})
print("Tasks loaded from file.")
else:
print("No existing task file found. Starting fresh.")
def save_tasks(self):
"""Save tasks to a file."""
with open(self.file_name, "w") as file:
for task in self.tasks:
file.write(f"{task['task']}|{task['priority']}\n")
print("Tasks saved to file.")
def add_task(self, task, priority=3):
"""Add a task to the to-do list with a specified priority (1-5)."""
if priority < 1 or priority > 5:
print("Invalid priority! Please choose a priority between 1 (highest) and 5 (lowest).")
else:
self.tasks.append({"task": task, "priority": priority})
self.tasks.sort(key=lambda x: x["priority"])
print(f"Task '{task}' with priority {priority} added.")
def remove_task(self, task_number):
"""Remove a task from the to-do list by its number."""
if 0 < task_number <= len(self.tasks):
removed_task = self.tasks.pop(task_number - 1)
print(f"Task '{removed_task['task']}' removed.")
else:
print("Invalid task number.")
def view_tasks(self):
"""View all tasks in the to-do list, sorted by priority."""
if not self.tasks:
print("No tasks in the list.")
else:
print("Your To-Do List (Sorted by Priority):")
for i, task in enumerate(self.tasks, 1):
print(f"{i}. {task['task']} [Priority: {task['priority']}]")
def clear_tasks(self):
"""Clear all tasks from the to-do list."""
confirm = input("Are you sure you want to clear all tasks? (y/n): ")
if confirm.lower() == 'y':
self.tasks.clear()
print("All tasks cleared.")
else:
print("Clear operation canceled.")
def main():
todo_list = ToDoList()
while True:
print("\n--- To-Do List Menu ---")
print("1. Add a task")
print("2. Remove a task")
print("3. View tasks")
print("4. Clear all tasks")
print("5. Save and Exit")
print("-----------------------")
choice = input("Enter your choice (1-5): ")
if choice == '1':
task = input("Enter the task: ")
priority = int(input("Enter the priority (1-5, with 1 being the highest): "))
todo_list.add_task(task, priority)
elif choice == '2':
todo_list.view_tasks()
task_number = int(input("Enter the task number to remove: "))
todo_list.remove_task(task_number)
elif choice == '3':
todo_list.view_tasks()
elif choice == '4':
todo_list.clear_tasks()
elif choice == '5':
todo_list.save_tasks()
print("Exiting the To-Do List app. Goodbye!")
break
else:
print("Invalid choice. Please enter a number between 1 and 5.")
if __name__ == "__main__":
main()
Step 2: Adding Functionality
In the ToDoList
class, we have defined several methods:
__init__
: Initializes the app and loads any existing tasks from a file.load_tasks
: Reads tasks from a file and populates the list.save_tasks
: Writes tasks to a file to ensure they persist between sessions.add_task
: Allows you to add new tasks with a priority level.remove_task
: Removes a specific task based on its number.view_tasks
: Displays all current tasks, sorted by priority.clear_tasks
: Clears all tasks from the list.
The main
function drives the app, presenting a menu for users to interact with. It allows you to add, remove, view, and clear tasks, as well as save and exit the app.
Step 3: Testing and Running Your App
- Save Your File: Make sure to save your Python file as
main.py
. - Run the Script: Open your terminal or command prompt, navigate to the directory where
main.py
is located, and run the script using the commandpython main.py
. - Interact with the App: Follow the on-screen prompts to add, remove, view, and manage tasks.
Conclusion:
Building a simple To-Do List App in Python is a great way to strengthen your understanding of basic programming concepts. Through this project, you’ve learned how to work with classes, handle user input, manage files for data persistence, and implement task prioritization. These are fundamental skills that lay the groundwork for more advanced Python projects.
This app can be expanded in countless ways, from adding a graphical interface to integrating more complex features like deadlines or reminders. The key takeaway is that even a simple project like this can teach you a lot about how to structure and organize code effectively.
As you continue to develop your coding skills, try experimenting with the code provided here. Modify it, add new features, and make it your own. Programming is all about creativity and problem-solving, and each project you complete brings you one step closer to mastering those skills.
Hope you enjoyed building Projects with us! Visit our Homepage and you get lots of projects Happy coding!